Cheating at z-depth sprite sorting for draw order
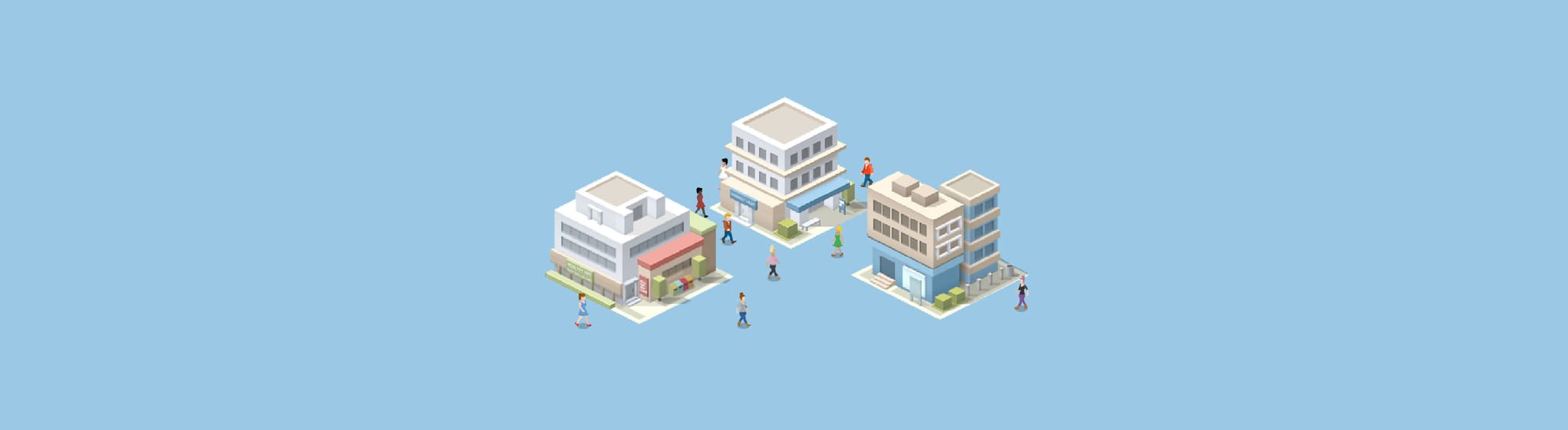
When implementing buildings in Pocket City, I discovered that ordering buildings was easy - just sort the z-index using the buildings's Y position whenever the buildings changed, then cache the buildings as a bitmap (combines a group of sprites into a single static sprite in Phaser).
However, when it came to sorting pedestrian sprites that walk around buildings, I had to recalculate the z-depth for both buildings and pedestrians on every update so that pedestrians would appear in front and behind each building appropriately. This meant recalculating structures and pedestrians on each update()
, and I could no longer cache structures as a single bitmap.
In a Cordova-based game, performance is a major concern, so I wondered it there was a any easy way to cheat and avoid the z-depth recalculation on every update.
I realized the building sprites could be divided into two sections - one that always appears behind pedestrians, and one that always appears on top.
I could layer my sprites into groups like so:
This meant splitting each building sprite into two sprites and stuffing the pedestrians layer in the middle. Each layer is a group that I add to my stage in the order that they should be drawn.
// In a parent Phaser.Group, I add each layer as a child.
// The layers will be drawn in the order they are added:
this.addChild(this.lowerStructureLayer);
this.addChild(this.pedestriansLayer);
this.addChild(this.upperStructureLayer);
Then, now that my pedestrians are in a separate layer, I can just call sort on the pedestrians without mixing in the structures.
// Sort on the pedestrian layer in the update() loop
pedestriansLayer.sort('y', Phaser.Group.SORT_ASCENDING);
And now that my structure layer is not being sorted, I can actually cache them as bitmap again!
this.lowerStructureLayer.cacheAsBitmap = true;
this.upperStructureLayer.cacheAsBitmap = true;
This strategy allowed me to save on z-index sorts and enabled caching the structures into two bitmaps (upper and lower). This exact solution may not be applicable to other games, but it shows that sometimes the solution is to cheat a bit and think outside the box!
The Result:
Note the pedestrians walking from the front to back of the building:
Bonus: Upper structure tinted for debugging: